Today we will see how to add days to a date object. Using the datetime.timedelta() method of datetime module you can add any number of days to a date object. Here is an example.
## Adding days to date in Python
import datetime
## Date object
date_original = datetime.date(2019, 7, 20)
## Days to add
days_to_add = 16
## Add
date_new = date_original + datetime.timedelta(days_to_add)
print("\n Original Date: ", date_original, "\n")
print("\n New Date: ", date_new, "\n")
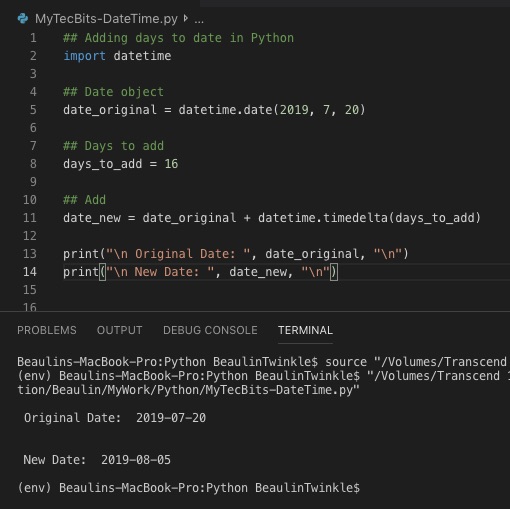
Reference
- About datetime.timedelta at Python Docs.
More Tips
- How to convert the date strings of mm/dd/yyyy and dd/mm/yyyy to date object in Python?
- How to convert seconds to days, hours, minutes and seconds in Python?