Sometime you may need to manually raise exception in Python programming instead of thrown automatically. For manually raising exception, you can use the raise statement. Here is an example of using raise.
try:
f = open('samples/myfile.txt')
s = f.readline()
f.close()
if len(s) > 0:
i = int(s.strip())
else:
raise ValueError("Manual exception - the file does not have any data.")
except (OSError, ValueError) as err:
print("\nError: {0}".format(err) , "\n")
except Exception as err:
print("\nSome Other Error: {0}".format(err), "\n")
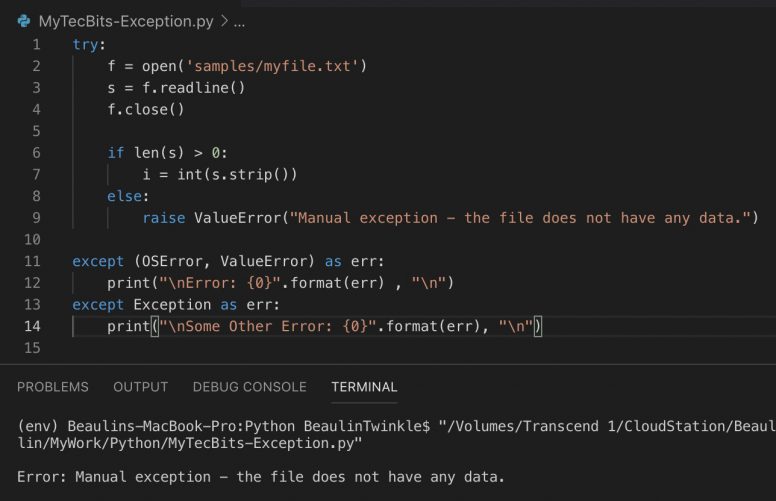
See my other article on catching multiple exceptions in an except clause.
Reference
- More about raising exceptions at Python docs.