There are multiple ways to merge two lists in Python. In my earlier article, we have seen merging two lists using the plus (+) operator and the list.extend() methods. In this article, let’s see how to concatenate two lists using the unpacking method and the sum() method.
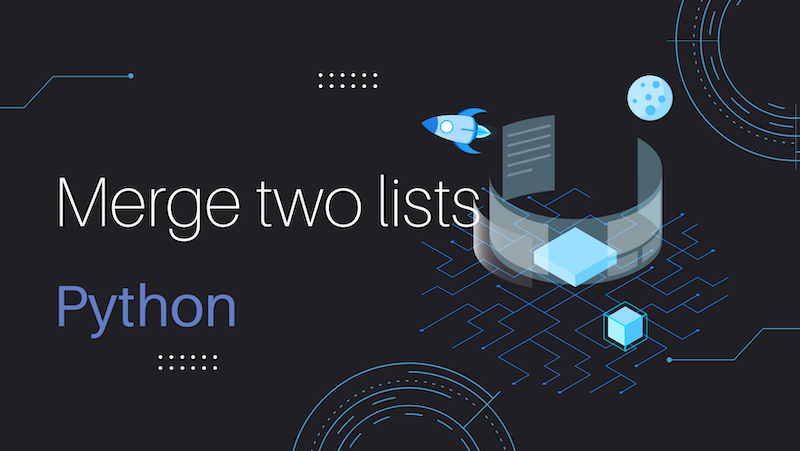
Using unpacking method with star (*) expression
One of the common way to concatenate or merge multiple lists is by using star (*) expression. Below is an example.
# Merging two lists using unpacking method
list_A = [100, 200, 300, 400]
list_B = [400, 1000, 2000]
list_Result = [*list_A, *list_B]
print(list_Result)
Result:
[100, 200, 300, 400, 400, 1000, 2000]
The advantage of using this unpacking method is, you can even merge lists with range and tuple. Here is an example of concatenating a list with a range.
# Merging a list and a range using unpacking method
list_A = [100, 200, 300, 400]
range_A = range(400, 1000, 100)
list_Result = [*list_A, *range_A]
print(list_Result)
Result:
[100, 200, 300, 400, 400, 500, 600, 700, 800, 900]
If you try to merge a list and a range using the plus (+) operator, you will get the error “TypeError: can only concatenate list (not “range”) to list“. In such situations, this unpacking method of merging will be helpful.
Using sum() function
Using the sum() function is a variation of the plus (+) operator to merge two lists. Here is an example.
# Merging two lists using sum
list_A = [100, 200, 300, 400]
list_B = [400, 1000, 2000]
list_Result = sum([list_A, list_B], [])
print(list_Result)
Result:
[100, 200, 300, 400, 400, 1000, 2000]
Merge two lists using addition assignment (+=)
Below is another way to use the plus (+) operator (actually addition assignment += ) to concatenate lists.
list_A = [100, 200, 300, 400]
list_B = [400, 1000, 2000]
list_A += list_B
print(list_A)
Result:
[100, 200, 300, 400, 400, 1000, 2000]
Hope this is helpful. In the next article we will see how to merge two or more dictionaries in Python.
Reference
- More about iterable unpacking at Python Docs.