String encoding is a fundamental aspect of modern programming, enabling the transformation of text into binary data that can be stored, transmitted, and processed by computers. In C#, developers have access to a wide range of encoding options, including the popular American Standard Code for Information Interchange (ASCII). In this article, we will see how to encode a string to ASCII in C#, with a practical example.
ASCII Encoding
ASCII encoding represents characters as numeric values, with each character assigned a unique number ranging from 0 to 127. This encoding scheme is primarily suited for the English language and includes commonly used characters like letters, digits, and basic punctuation marks.
Encoding a String to ASCII in C#
To encode a string to ASCII in C#, developers can leverage the built-in Encoding.ASCII property found in the System.Text namespace. Let’s walk through an example:
using System;
using System.Text;
public class Program
{
public static void Main()
{
string inputString = "Hello, MyTecBits!";
byte[] asciiByteArray = Encoding.ASCII.GetBytes(inputString);
string asciiEncoded = String.Join(" ", asciiByteArray);
Console.WriteLine("ASCII encoded value: ");
Console.WriteLine(asciiEncoded);
}
}
Result:
ASCII-encoded bytes:
72 101 108 108 111 44 32 77 121 84 101 99 66 105 116 115 33
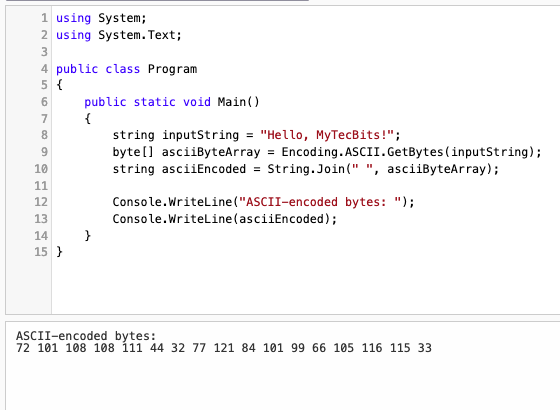
In this example, we first import the required namespaces, including System.Text. We define the input string, Hello, MyTecBits! and create a byte array asciiByteArray using the Encoding.ASCII.GetBytes() method to encode the input string. Finally, we join the elements of the byte array into a string and display the ASCII encoded value.
You can check our online ASCII Encoder Tool here. We have used the above c# code to develop this tool. It will encode the given string to ASCII format.
Related Article
Reference
- Read more about Encoding.ASCII property at Microsoft Docs.